- Literate Programming made easy
- Create live documents in Markdown
- Program in any language you like
- Use your favourite editor/IDE
- Works well with version control
Literate programming /ˈlɪtəɹət ˈpɹəʊɡɹæmɪŋ/ (computing) Literate programming is a programming paradigm introduced by Donald Knuth in which a program is given as an explanation of the program logic in a natural language, such as English, interspersed with snippets of macros and traditional source code, from which a compilable source code can be generated. Wikipedia
About
Entangled helps you write Literate Programs in Markdown. You put all your code inside Markdown code blocks. Entangled automatically extracts the code and writes it to more traditional source files. You can then edit these generated files, and the changes are being fed back to the Markdown.
We’re trying to increase the visibility of Entangled. If you like
Entangled, please consider adding this badge to the appropriate location in your
project:
[](https://entangled.github.io/)
Get Started
With the 2.0 release, Entangled is now available as a Python package,
installable through pip
,
pip install entangled_cli
Write Markdown
“A critical aspect of a programming language is the means it provides for using names to refer to computational objects.” Abelson, Sussman & Sussman - SICP
Name your code
The square of the hypothenuse is the sum
of the two right-angled sides squared:
$$a^2 + b^2 = c^2$$
``` {.python #pythagoras}
def vector_length(x, y):
return sqrt(x**2 + y**2)
```
The square of the hypothenuse is the sum of the two right-angled sides squared: \[a^2 + b^2 = c^2\]
def vector_length(x, y):
return sqrt(x**2 + y**2)
“Let us change our traditional attitude to the construction of programs: Instead of imagining that our main task is to instruct a computer what to do, let us concentrate rather on explaining to human beings what we want a computer to do.” Knuth - Literate Programming
Compose your program
To count the words in a sentence, first
split the sentence into words, then
count the number of words in the list.
``` {.python #word-count}
def word_count(sentence):
<<split-into-words>>
<<count-words>>
return count
```
`.split`
The default arguments to the
method split on any white space.
``` {.python #split-into-words}
words = sentence.split()
```
`length`
Counting is done with the
function.
``` {.python #count-words}
count = len(words)
```
To count the words in a sentence, first split the sentence into words, then count the number of words in the list.
def word_count(sentence):
<<split-into-words>>
<<count-words>>
return count
The default arguments to the .split
method split on any
white space.
= sentence.split() words
Counting is done with the len
function.
= len(words) count
“Talk is cheap. Show me the code.” Linus Torvalds
Documentation
Bootstrap web template for Pandoc
To help you easily create a presentable website from your literate code, we provide a Bootstrap template for Pandoc. Probably the best way to use this template, is to fork our repository at entangled/bootstrap-submodule, and add your fork as a submodule in your project:
git submodule add git@github.com:<my bootstrap-submodule fork>
This way you can tweak the template to your own wishes. If you want to play around first, you can also use the cookiecutter template,
cookiecutter https://github.com/entangled/bootstrap
but this approach is less flexible. For more information, see the tutorial.
External Links
Blogs
- Why all you’ll ever need is Markdown — Introduction to Pandoc, and Pandoc filters.
- Entangled, a bi-directional Literate Programming tool — Presenting Entangled, a tool for pain free literate programming.
- Literate Programming in Science: the why — Explaining why Literate Programming is particularly well matched with scientific programming.
- Literate Programming in Science: the how — Showing methods for Literate Programming, comparing Entangled to Jupyter, RMarkdown and Org-mode.
- Unweaving legacy code using Entangled — Showing how you can use Entangled to reverse-engineer unreadable legacy code.
Literate Books
These are some awesome books written with a literate philosophy in mind.
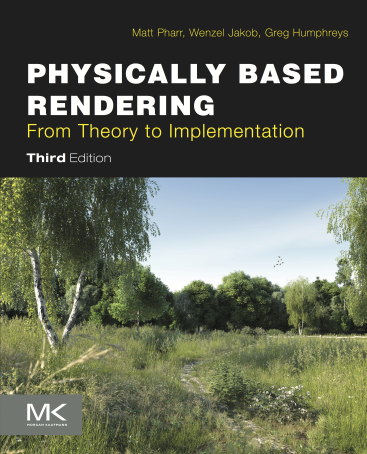
Pharr, Jakob & Humphreys - Physically Based Rendering
Explains physically realistic 3D rendering, while implementing the same techniques in C++. This book is so amazing, it actually won an Acadamy Award for technical achievement. The book uses the same noweb notation for code block references we do.
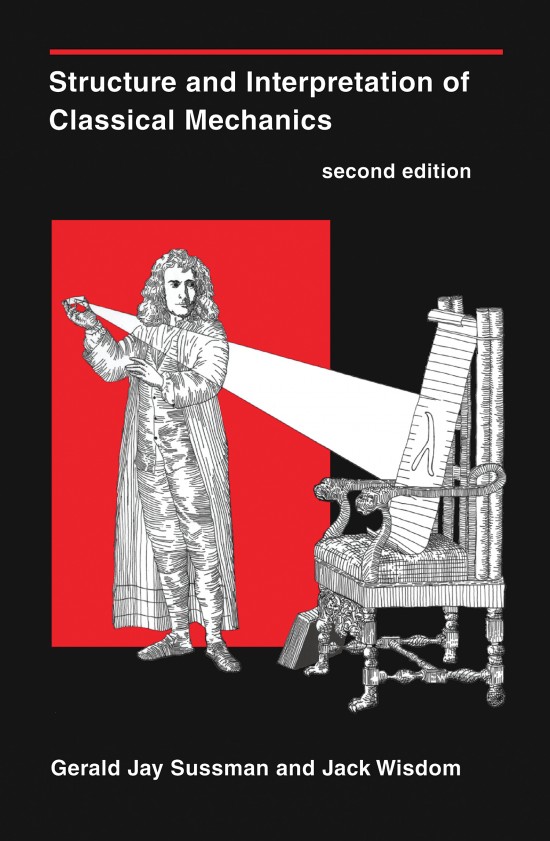
Sussman & Wisdom - Structure and Interpretation of Classical Mechanics
Does not use noweb, but subscribes to the many founding principles of literate programming. This is a text book on classical mechanics and specifically the Lagrangian and Hamiltonian descriptions of physics.
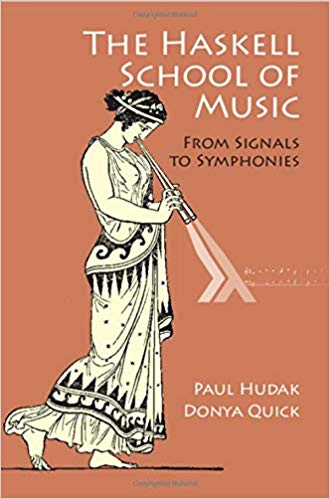
Hudak & Quick - The Haskell School of Music
From signals to symphonies, this book fuses the authors’ passion for music and the Haskell programming language.
Pandoc filters
- Knitty — Expands code-cells through a Jupyter interface. Uses Panflute.
- pandocsql — Inserts tables in your markdown into an Sqlite database, and run queries that appear as tables in the output. Uses Panflute.
Dev tools
- Panflute — A “Pythonic” interface for creating Pandoc filters.